In my recent job search, I have been interviewed at many companies, In one of the interviews, the Interviewer asked me to create a TicTacToe Game. The interviewer might expect you to solve this in React JS or React Native based on the role that you are being interviewed for. This question is even mentioned in React Native Documentation – React Official Documentation – TicTacToe
Let’s start by setting up the game board. We’ll create a 3×3 grid where players can place their moves. Here, we are creating the 3X3 grid a little differently than the way mentioned in the documentation.
const TicTacToe = () => {
const [board, setBoard] = useState(Array(9).fill(null));
const renderSquare = (index) => (
<button className="square" onClick={() => handleClick(index)}>
{board[index]}
</button>
);
return (
<div className="game">
<div className="board">
{board.map((_, index) => renderSquare(index))}
</div>
</div>
);
};
.game {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #282c34;
color: white;
font-family: 'Arial', sans-serif;
}
.board {
display: flex;
flex-wrap: wrap;
width: 300px;
}
.square {
width: 100px;
height: 100px;
display: flex;
align-items: center;
justify-content: center;
font-size: 48px;
border: 1px solid #ddd;
background-color: #444;
color: white;
cursor: pointer;
}
For every click, we need to check if that is a winner-deciding click. To decide the winner, we will call calculateWinner
. Here, lines
contains different possibilities for winning. if [0th, 1st, 2nd]
index square boxes contain the same (X or O) then it’s a winner possibility. Similarly, the other lines
elements.
const calculateWinner = (squares) => {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] ===
squares[c]) {
return squares[a];
}
}
return null;
};
You can check the lines
elements from this image below.
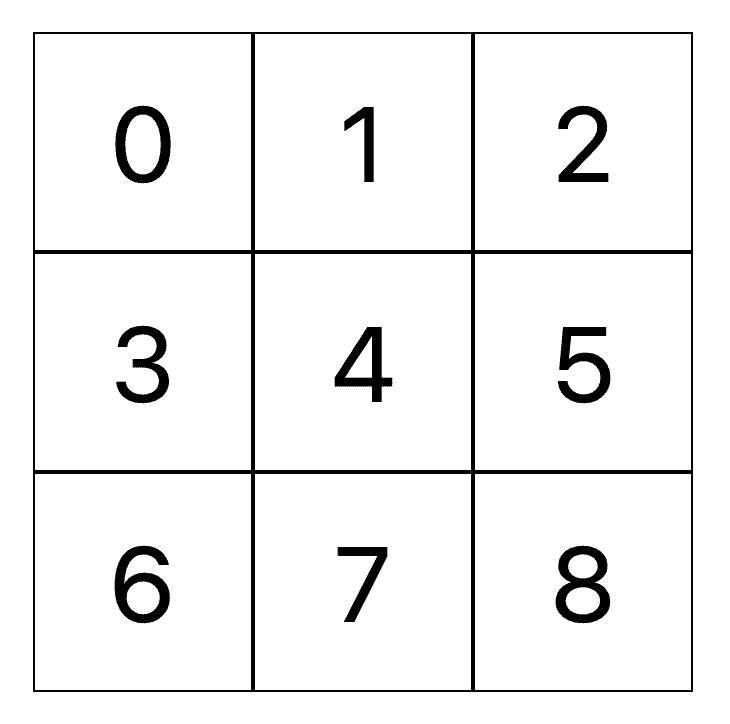
Here is the total code for the TicTacToe game.
import React, { useState } from 'react';
import './TicTacToe.css';
const TicTacToe = () => {
const [board, setBoard] = useState(Array(9).fill(null));
const [isXNext, setIsXNext] = useState(true);
const handleClick = (index) => {
if (board[index] || calculateWinner(board)) return;
const newBoard = board.slice();
newBoard[index] = isXNext ? 'X' : 'O';
setBoard(newBoard);
setIsXNext(!isXNext);
};
const renderSquare = (index) => (
<button className="square" onClick={() => handleClick(index)}>
{board[index]}
</button>
);
const winner = calculateWinner(board);
const status = winner ? `Winner: ${winner}` : `Next player: ${isXNext ? 'X' : 'O'}`;
return (
<div className="game">
<div className="status">{status}</div>
<div className="board">
{board.map((_, index) => renderSquare(index))}
</div>
</div>
);
};
const calculateWinner = (squares) => {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] ===
squares[c]) {
return squares[a];
}
}
return null;
};
export default TicTacToe;
.game {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #282c34;
color: white;
font-family: 'Arial', sans-serif;
}
.status {
margin-bottom: 20px;
font-size: 24px;
}
.board {
display: flex;
flex-wrap: wrap;
width: 300px;
}
.square {
width: 100px;
height: 100px;
display: flex;
align-items: center;
justify-content: center;
font-size: 48px;
border: 1px solid #ddd;
background-color: #444;
color: white;
cursor: pointer;
}
By following these steps, you’ll have a fully functional TicTacToe game in React.