You will be given a number, and need to prepare N X N boxes on the screen, clicking on it should show a blue colour. While Preparing we need to create a single box first then we need to build the N X N Box grid. This question was asked in Kotak‘s interview first round, where the interviewer was from the BarRaiser. As I was being interviewed for a React Native role in Kotak, I preferred writing the code in React Native itself.
We’ll start by creating a reusable Box component that represents each box in our grid. This component will accept props for colour and onPress event handling. Here’s the code for the Box component:
// Box component
const Box = ({ color, onPress }) => {
return (
<TouchableOpacity onPress={onPress}>
<View style={[styles.box, { backgroundColor: color }]} />
</TouchableOpacity>
);
};
Now, we will be using this Box component, and showing the N X N Grid of Boxes, where N rows, N Columns will be present as below.

We’ll create a parent component called GridOfBoxes that renders an N x N grid of Box components. We’ll use the state to manage the colour of each box and handle box clicks to toggle their colours. Here’s the code for the GridOfBoxes component:
// Parent component
const GridOfBoxes = ({ size }) => {
const [boxColors, setBoxColors] = useState(Array(size * size).fill("white"));
const handleBoxPress = (index) => {
const newBoxColors = [...boxColors];
newBoxColors[index] = newBoxColors[index] === "white" ? "blue" : "white";
setBoxColors(newBoxColors);
};
return (
<View style={styles.container}>
{Array.from({ length: size }, (_, rowIndex) => (
<View key={rowIndex} style={styles.row}>
{Array.from({ length: size }, (_, colIndex) => {
const index = rowIndex * size + colIndex;
return (
<Box
key={index}
color={boxColors[index]}
onPress={() => handleBoxPress(index)}
/>
);
})}
</View>
))}
</View>
);
};
const styles = StyleSheet.create({
container: {
flexDirection: "column",
alignItems: "center",
backgroundColor: "black",
},
row: {
flexDirection: "row",
},
box: {
width: 50,
height: 50,
margin: 5,
},
});
export default GridOfBoxes;
When we click on any box then it will be turning to blue as per the problem statement.
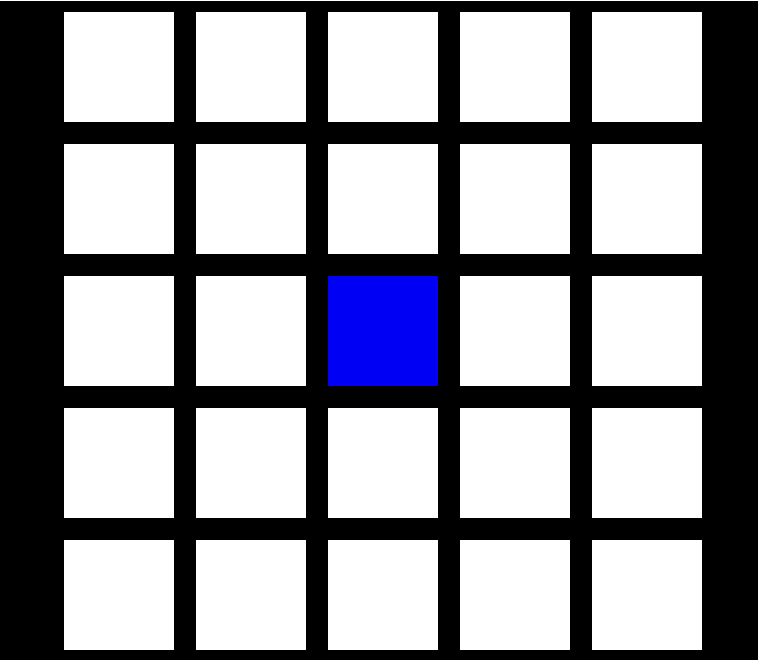
1 comment
[…] Here is the link to the blog post: https://rowdycoders.com/building-an-n-x-n-grid-of-boxes-in-react-kotak-interview […]